それぞれ別のSQLで取得したListを結合して
昇順に並び替える方法を紹介していきます。
SQLで条件を指定し結合することも可能ですが、
どうしても複雑になってしまいがちです。
また改修する場合、
最初にソースを作成した人が
改修することは、ほぼありません。
したがって複雑なSQLを作成すると
改修者にとっては、とても大変で
デグレを起こす原因にもなります。
基本的に推奨されるソースというのは、
Service class(DBからsqlで情報を取得した想定のクラス)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 |
package comp; import java.sql.Timestamp; public class Service { //ユーザID private int serviceUserId = 0; //名前 private String serviceUserName = ""; //処理日時 private Timestamp serviceActionDate = null; public int getServiceUserId() { return serviceUserId; } public void setServiceUserId(int serviceUserId) { this.serviceUserId = serviceUserId; } public String getServiceUserName() { return serviceUserName; } public void setServiceUserName(String serviceUserName) { this.serviceUserName = serviceUserName; } public Timestamp getServiceActionDate() { return serviceActionDate; } public void setServiceActionDate(Timestamp serviceActionDate) { this.serviceActionDate = serviceActionDate; } /*データの作成 * 概要:sqlで取得した想定のデータを作成する */ public Service(int num) { this.serviceUserId = num; this.serviceUserName = "user" + num; //タイムスタンプをランダムに作成 long offset = Timestamp.valueOf("2020-01-01 00:00:00").getTime(); long end = Timestamp.valueOf("2021-01-01 00:00:00").getTime(); long diff = end - offset + 1; Timestamp randTime = new Timestamp(offset + (long) (Math.random() * diff)); this.serviceActionDate = randTime; } //検索条件の取得結果(SQLで取得した想定のメソッド) public static List<Service> search(int num) { List<Service> list = new ArrayList<Service>(); int cnt = 5; for (int i = num; i < cnt + num; i++) { Service sqlVal = new Service(i); list.add(sqlVal); } return list; } } |
ValueSample class(データ格納、読み出しのDTOクラス)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 |
package comp; import java.sql.Timestamp; public class ValueSample { //ユーザID private int userId = 0; //名前 private String userName = ""; //処理日時 private Timestamp actionDate = null; public int getUserId() { return userId; } public void setUserId(int userId) { this.userId = userId; } public String getUserName() { return userName; } public void setUserName(String userName) { this.userName = userName; } public Timestamp getActionDate() { return actionDate; } public void setActionDate(Timestamp actionDate) { this.actionDate = actionDate; } } |
CompSample class(ソート実行のmainクラス)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 |
package comp; import java.util.ArrayList; import java.util.Comparator; import java.util.List; public class CompSample { public static void main(String[] args) { //箱を用意 List<ValueSample> aEntityList = new ArrayList<ValueSample>(); List<ValueSample> bEntityList = new ArrayList<ValueSample>(); List<ValueSample> allList = new ArrayList<ValueSample>(); //検索条件の取得結果A(SQLで取得した想定) List<Service> aList = Service.search(1); //検索条件の取得結果B(SQLで取得した想定) List<Service> bList = Service.search(6); //aListの件数分繰り返す for (Service row : aList) { ValueSample val = new ValueSample(); val.setUserId(row.getServiceUserId()); val.setUserName(row.getServiceUserName()); val.setActionDate(row.getServiceActionDate()); aEntityList.add(val); } //bListの件数分繰り返す for (Service row : bList) { ValueSample val = new ValueSample(); val.setUserId(row.getServiceUserId()); val.setUserName(row.getServiceUserName()); val.setActionDate(row.getServiceActionDate()); bEntityList.add(val); } allList.addAll(aEntityList); allList.addAll(bEntityList); /*Java 7 以前 // //ソートの処理 // Comparator<ValueSample> comparator = new Comparator<ValueSample>() { // public int compare(ValueSample o1, ValueSample o2) { // //昇順で日付を比較 // return o1.getActionDate().compareTo(o2.getActionDate()); // } // }; // //実行している間、アクセスされないようにロック処理 // synchoronized(allList){ // //日付の昇順でソート実行 // Collection.sort(allList,comparator); // } */ //Java 8 以降(日付の昇順) allList.sort(Comparator.comparing(ValueSample::getActionDate)); //Java 8 以降(日付の降順) //allList.sort(Comparator.comparing(ValueSample::getActionDate).reversed()); //コンソール出力用 for (ValueSample row : allList) { System.out.print(row.getUserId() + "\t"); System.out.print(row.getUserName() + "\t"); System.out.println(row.getActionDate().toString()); } } } |
コンソール出力結果
1 2 3 4 5 6 7 8 9 10 |
4 user4 2020-01-02 12:36:44.095 9 user9 2020-01-09 07:33:18.472 10 user10 2020-01-23 07:47:31.763 8 user8 2020-02-23 02:58:36.296 5 user5 2020-05-06 02:10:14.742 7 user7 2020-06-09 02:37:04.757 3 user3 2020-08-23 21:01:22.492 1 user1 2020-08-28 06:38:15.655 6 user6 2020-09-26 13:13:31.231 2 user2 2020-11-16 14:29:35.341 |
Java 8以降は、
Listにsort(Comparator)が
追加されたので、
シンプルなコードで
昇順、降順のソートが可能です。
ソート条件は
Comparator.comparing
(ValueSample::getActionDate)
ソートキー Function を受け取り、
ソートキーを含む型の Comparator を返却。
それぞれ別のSQLで取得したListを結合して
昇順に並び替える方法を解説してきました。
今の年収に満足していますか?
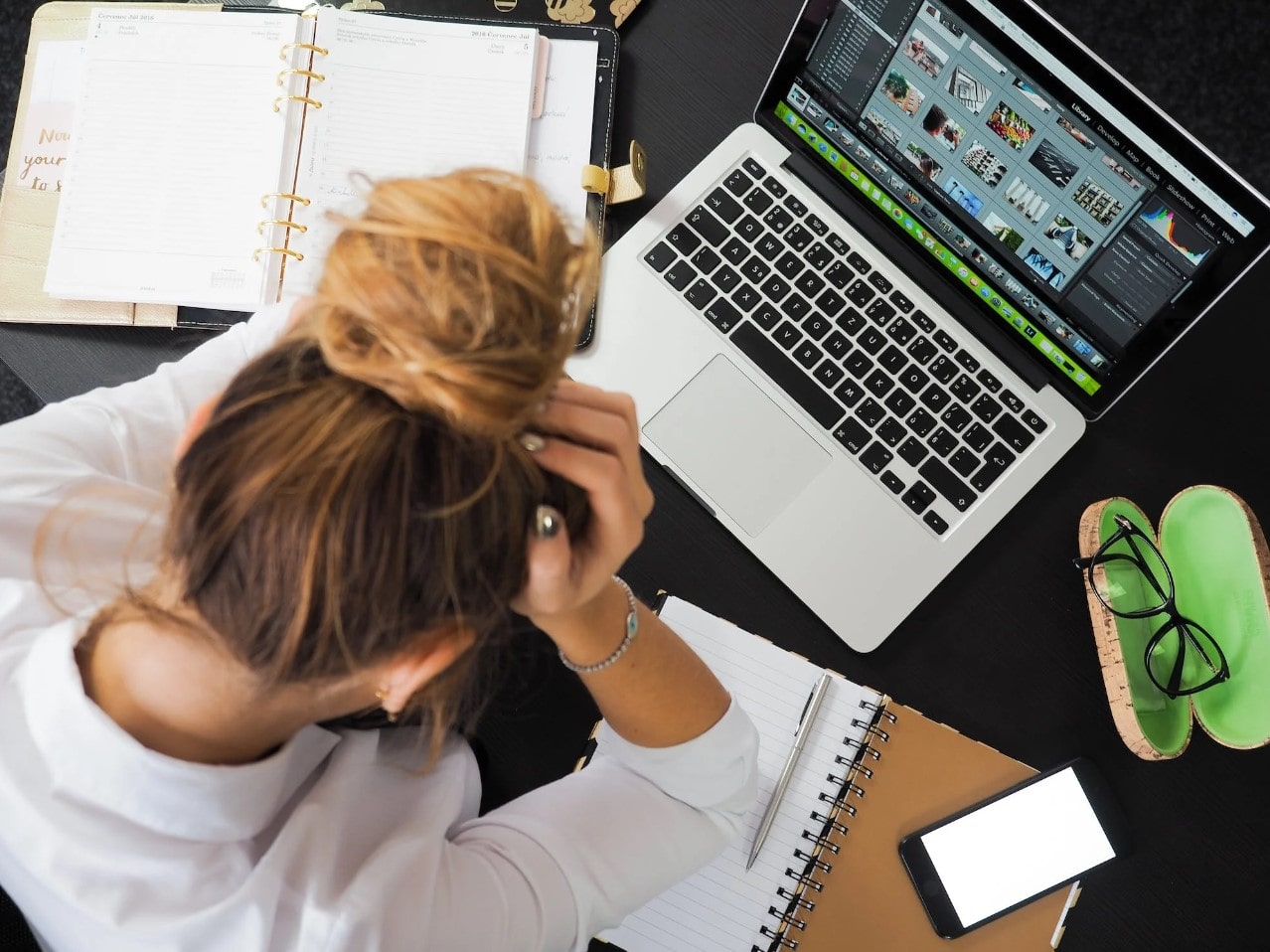
同じ契約金で業務委託していても
在籍している会社によって給料が違います。
今と同じスキル・業務内容でも
年収が変わるということです。
さらに以下を考慮すると
より年収アップにつながります。
- スキルセットと需要
- 経験と実績
- 業界や地域の市場価値
- 転職時の交渉スキル
転職先の企業の給与体系や
業界の標準的な給与水準を調査し、
給与のみでなく、
福利厚生やキャリア成長の機会、
ワークライフバランスなどの要素も
総合的に考慮することが重要です。
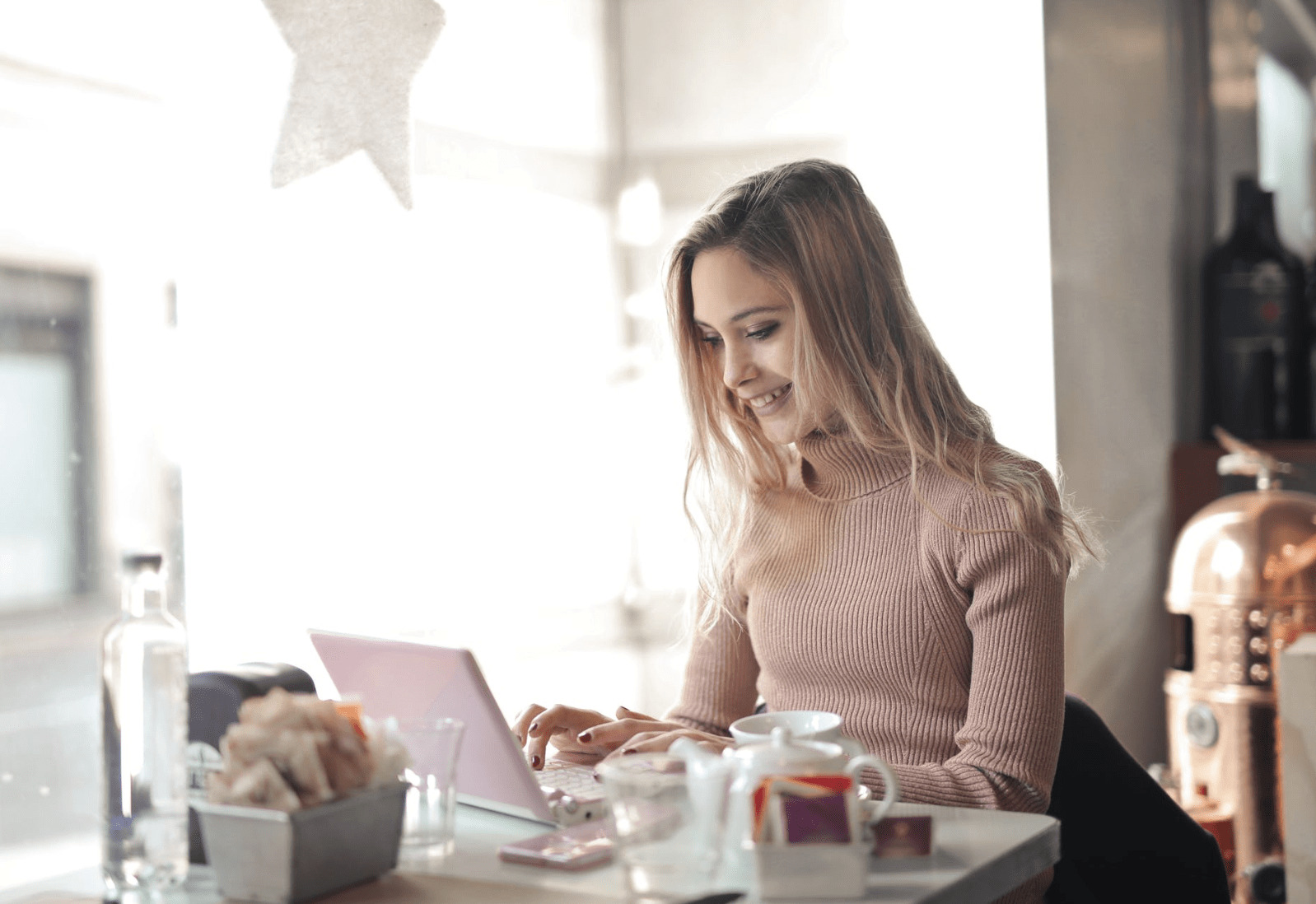
Kindle Unlimitedは、Amazonが提供する
月額定額制の電子書籍サービスを活用します。
また、Kindle Unlimitedには、
体験版が提供されています。
体験版では、一定期間(通常は30日間)に限り、
Kindle Unlimitedのサービスを
無料で利用することができます。
1.豊富な書籍のラインナップ
Kindle Unlimitedには、幅広いジャンルの書籍が
数十万冊以上含まれています。
小説、ビジネス書、自己啓発書、
学術書、漫画など、
様々なテーマや興味に合わせて
多彩な選択肢があります。
2.サービスの内容を体験
体験版では、Kindle Unlimitedの全ての機能を使いながら、
豊富な書籍のラインナップや
読書体験を体験することができます。
自身の読書の好みや
利用頻度に合うかどうかを確認できます。
3.解約が可能
体験版期間中にKindle Unlimitedのサービスに
満足しなかった場合、
期限内に解約することができます。
その場合、追加料金はかかりません。

Kindle Unlimitedでは
さまざまなジャンルの書籍が提供されています。
ITエンジニアとしてスキルを向上させたり
新しい技術を学んだりする際に、
Kindle Unlimitedは
便利な資源となるのでおすすめです。
この記事へのコメントはありません。